14 Most used realtime business use case for Plugin scenario based snippets in Dataverse/ Dynamics 365
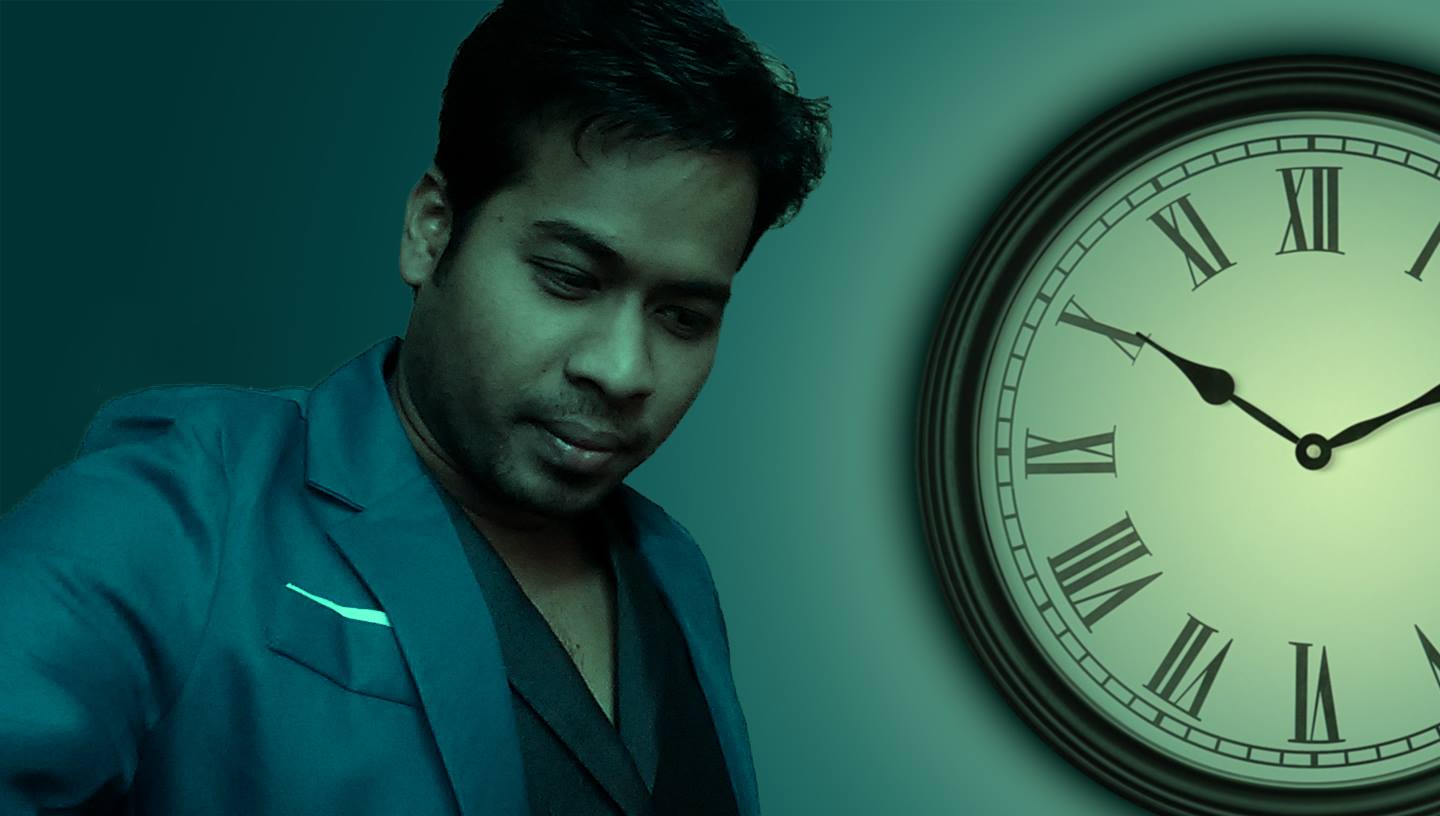
Here is a list of sample Plugin Scenarios we mostly use in real live projects
1. Automatically generate a unique account number when a new account is created.
Scenario: Automatically generate a unique account number when a new account is created.
Explanation: This plugin triggers on the creation of a new account and assigns a unique account number based on the current timestamp.
public class AccountNumberPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity account = (Entity)context.InputParameters["Target"];
if (account.LogicalName == "account")
{
// Logic to generate unique account number
account["accountnumber"] = "ACC" + DateTime.Now.Ticks.ToString();
}
}
}
}
2. Email Validation Server Side
Scenario: Validate the email format of a contact before saving.
Explanation: This plugin checks the format of the email address of a contact. If the format is invalid, it throws an exception to prevent saving.
public class EmailValidationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity contact = (Entity)context.InputParameters["Target"];
if (contact.LogicalName == "contact" && contact.Attributes.Contains("emailaddress1"))
{
string email = contact["emailaddress1"].ToString();
if (!Regex.IsMatch(email, @"^[^@\s]+@[^@\s]+\.[^@\s]+$"))
{
throw new InvalidPluginExecutionException("Invalid email format.");
}
}
}
}
}
3. Opportunity Probability Update
Scenario: Update opportunity probability based on stage.
public class OpportunityProbabilityPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity opportunity = (Entity)context.InputParameters["Target"];
if (opportunity.LogicalName == "opportunity" && opportunity.Attributes.Contains("stageid"))
{
Guid stageId = (Guid)opportunity["stageid"];
// Example mapping of stage IDs to probabilities
Dictionary<Guid, int> stageProbabilityMapping = new Dictionary<Guid, int>
{
{ new Guid("00000000-0000-0000-0000-000000000001"), 10 },
{ new Guid("00000000-0000-0000-0000-000000000002"), 30 },
{ new Guid("00000000-0000-0000-0000-000000000003"), 60 },
{ new Guid("00000000-0000-0000-0000-000000000004"), 90 }
};
if (stageProbabilityMapping.ContainsKey(stageId))
{
opportunity["closeprobability"] = stageProbabilityMapping[stageId];
}
}
}
}
}
Explanation: This plugin sets the probability of closing an opportunity based on the current sales stage.
4. Duplicate Detection
Scenario: Prevent the creation of duplicate records.
public class DuplicateDetectionPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity entity = (Entity)context.InputParameters["Target"];
if (entity.LogicalName == "account" && entity.Attributes.Contains("name"))
{
string accountName = entity["name"].ToString();
QueryExpression query = new QueryExpression("account")
{
ColumnSet = new ColumnSet("name")
};
query.Criteria.AddCondition("name", ConditionOperator.Equal, accountName);
EntityCollection results = service.RetrieveMultiple(query);
if (results.Entities.Count > 0)
{
throw new InvalidPluginExecutionException("An account with this name already exists.");
}
}
}
}
}
Explanation: This plugin checks for existing accounts with the same name to prevent duplicates.
5. Case Escalation
Scenario: Escalate cases that have not been resolved within a certain time frame.
public class CaseEscalationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity caseEntity = (Entity)context.InputParameters["Target"];
if (caseEntity.LogicalName == "incident" && caseEntity.Attributes.Contains("createdon"))
{
DateTime createdOn = (DateTime)caseEntity["createdon"];
if (DateTime.UtcNow - createdOn > TimeSpan.FromDays(3))
{
caseEntity["statuscode"] = new OptionSetValue(2); // Example status for escalation
}
}
}
}
}
Explanation: This plugin escalates cases that have not been resolved within three days by changing their status.
6. Invoice Due Date Calculation
Scenario: Automatically calculate and set the due date on an invoice
public class InvoiceDueDatePlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity invoice = (Entity)context.InputParameters["Target"];
if (invoice.LogicalName == "invoice" && invoice.Attributes.Contains("createdon"))
{
DateTime createdOn = (DateTime)invoice["createdon"];
invoice["duedate"] = createdOn.AddDays(30); // Example due date of 30 days after creation
}
}
}
}
Explanation: This plugin sets the due date of an invoice to 30 days after the creation date.
7. Lead Qualification
Scenario: Automatically qualify leads based on criteria. Explanation: This plugin qualifies leads with a budget amount greater than $10,000.
public class LeadQualificationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity lead = (Entity)context.InputParameters["Target"];
if (lead.LogicalName == "lead" && lead.Attributes.Contains("budgetamount"))
{
Money budget = (Money)lead["budgetamount"];
if (budget.Value > 10000) // Example qualification criteria
{
lead["statecode"] = new OptionSetValue(1); // Qualified
lead["statuscode"] = new OptionSetValue(3); // Example status for qualified
}
}
}
}
}
8. Order Total Calculation
Scenario: Calculate the total amount of an order based on line items.
public class OrderTotalCalculationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity order = (Entity)context.InputParameters["Target"];
if (order.LogicalName == "salesorder")
{
QueryExpression query = new QueryExpression("salesorderdetail")
{
ColumnSet = new ColumnSet("extendedamount")
};
query.Criteria.AddCondition("salesorderid", ConditionOperator.Equal, order.Id);
EntityCollection orderDetails = service.RetrieveMultiple(query);
decimal totalAmount = 0;
foreach (Entity detail in orderDetails.Entities)
{
if (detail.Attributes.Contains("extendedamount"))
{
totalAmount += ((Money)detail["extendedamount"]).Value;
}
}
order["totalamount"] = new Money(totalAmount);
}
}
}
}
Explanation: This plugin calculates the total amount of an order by summing up the extended amounts of all related order details.
9. Task Creation on Case Creation
Scenario: Automatically create a follow-up task when a case is created.
public class CreateTaskOnCaseCreation : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity caseEntity = (Entity)context.InputParameters["Target"];
if (caseEntity.LogicalName == "incident")
{
Entity task = new Entity("task");
task["subject"] = "Follow up on case: " + caseEntity["title"];
task["scheduledstart"] = DateTime.Now.AddDays(1);
task["scheduledend"] = DateTime.Now.AddDays(1);
task["regardingobjectid"] = new EntityReference("incident", caseEntity.Id);
service.Create(task);
}
}
}
}
Explanation: This plugin creates a follow-up task with a due date one day after the creation of a new case.
10. Contact Birthday Reminder
Scenario: Send a reminder email when a contact’s birthday is approaching. Explanation: This plugin sends an email reminder one week before a contact’s birthday.
public class BirthdayReminderPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity contact = (Entity)context.InputParameters["Target"];
if (contact.LogicalName == "contact" && contact.Attributes.Contains("birthdate"))
{
DateTime birthDate = (DateTime)contact["birthdate"];
if (birthDate.Month == DateTime.Now.Month && birthDate.Day == DateTime.Now.Day + 7)
{
Entity email = new Entity("email");
email["subject"] = "Upcoming Birthday Reminder";
email["description"] = "Reminder: " + contact["fullname"] + "'s birthday is coming up in a week.";
email["to"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("contact", contact.Id) } });
email["from"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("systemuser", context.UserId) } });
service.Create(email);
}
}
}
}
}
11. Lead Scoring
Scenario: Automatically score leads based on attributes. Explanation: This plugin assigns a score to a lead based on their budget amount and industry code.
public class LeadScoringPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity lead = (Entity)context.InputParameters["Target"];
if (lead.LogicalName == "lead")
{
int score = 0;
if (lead.Attributes.Contains("budgetamount") && ((Money)lead["budgetamount"]).Value > 5000)
{
score += 10;
}
if (lead.Attributes.Contains("industrycode") && (OptionSetValue)lead["industrycode"] == new OptionSetValue(1))
{
score += 20;
}
lead["leadscore"] = score;
}
}
}
}
Here are 20 commonly used scenario-based plugin sample codes with explanations for Dynamics 365/Dataverse that address real-time business use cases:
1. Account Number Auto-Generation
Scenario: Automatically generate a unique account number when a new account is created.
csharpCopy codepublic class AccountNumberPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity account = (Entity)context.InputParameters["Target"];
if (account.LogicalName == "account")
{
// Logic to generate unique account number
account["accountnumber"] = "ACC" + DateTime.Now.Ticks.ToString();
}
}
}
}
Explanation: This plugin triggers on the creation of a new account and assigns a unique account number based on the current timestamp.
2. Email Validation
Scenario: Validate the email format of a contact before saving.
csharpCopy codepublic class EmailValidationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity contact = (Entity)context.InputParameters["Target"];
if (contact.LogicalName == "contact" && contact.Attributes.Contains("emailaddress1"))
{
string email = contact["emailaddress1"].ToString();
if (!Regex.IsMatch(email, @"^[^@\s]+@[^@\s]+\.[^@\s]+$"))
{
throw new InvalidPluginExecutionException("Invalid email format.");
}
}
}
}
}
Explanation: This plugin checks the format of the email address of a contact. If the format is invalid, it throws an exception to prevent saving.
3. Opportunity Probability Update
Scenario: Update opportunity probability based on stage.
csharpCopy codepublic class OpportunityProbabilityPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity opportunity = (Entity)context.InputParameters["Target"];
if (opportunity.LogicalName == "opportunity" && opportunity.Attributes.Contains("stageid"))
{
Guid stageId = (Guid)opportunity["stageid"];
// Example mapping of stage IDs to probabilities
Dictionary<Guid, int> stageProbabilityMapping = new Dictionary<Guid, int>
{
{ new Guid("00000000-0000-0000-0000-000000000001"), 10 },
{ new Guid("00000000-0000-0000-0000-000000000002"), 30 },
{ new Guid("00000000-0000-0000-0000-000000000003"), 60 },
{ new Guid("00000000-0000-0000-0000-000000000004"), 90 }
};
if (stageProbabilityMapping.ContainsKey(stageId))
{
opportunity["closeprobability"] = stageProbabilityMapping[stageId];
}
}
}
}
}
Explanation: This plugin sets the probability of closing an opportunity based on the current sales stage.
4. Duplicate Detection
Scenario: Prevent the creation of duplicate records.
csharpCopy codepublic class DuplicateDetectionPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity entity = (Entity)context.InputParameters["Target"];
if (entity.LogicalName == "account" && entity.Attributes.Contains("name"))
{
string accountName = entity["name"].ToString();
QueryExpression query = new QueryExpression("account")
{
ColumnSet = new ColumnSet("name")
};
query.Criteria.AddCondition("name", ConditionOperator.Equal, accountName);
EntityCollection results = service.RetrieveMultiple(query);
if (results.Entities.Count > 0)
{
throw new InvalidPluginExecutionException("An account with this name already exists.");
}
}
}
}
}
Explanation: This plugin checks for existing accounts with the same name to prevent duplicates.
5. Case Escalation
Scenario: Escalate cases that have not been resolved within a certain time frame.
csharpCopy codepublic class CaseEscalationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity caseEntity = (Entity)context.InputParameters["Target"];
if (caseEntity.LogicalName == "incident" && caseEntity.Attributes.Contains("createdon"))
{
DateTime createdOn = (DateTime)caseEntity["createdon"];
if (DateTime.UtcNow - createdOn > TimeSpan.FromDays(3))
{
caseEntity["statuscode"] = new OptionSetValue(2); // Example status for escalation
}
}
}
}
}
Explanation: This plugin escalates cases that have not been resolved within three days by changing their status.
6. Invoice Due Date Calculation
Scenario: Automatically calculate and set the due date on an invoice.
csharpCopy codepublic class InvoiceDueDatePlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity invoice = (Entity)context.InputParameters["Target"];
if (invoice.LogicalName == "invoice" && invoice.Attributes.Contains("createdon"))
{
DateTime createdOn = (DateTime)invoice["createdon"];
invoice["duedate"] = createdOn.AddDays(30); // Example due date of 30 days after creation
}
}
}
}
Explanation: This plugin sets the due date of an invoice to 30 days after the creation date.
7. Lead Qualification
Scenario: Automatically qualify leads based on criteria.
csharpCopy codepublic class LeadQualificationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity lead = (Entity)context.InputParameters["Target"];
if (lead.LogicalName == "lead" && lead.Attributes.Contains("budgetamount"))
{
Money budget = (Money)lead["budgetamount"];
if (budget.Value > 10000) // Example qualification criteria
{
lead["statecode"] = new OptionSetValue(1); // Qualified
lead["statuscode"] = new OptionSetValue(3); // Example status for qualified
}
}
}
}
}
Explanation: This plugin qualifies leads with a budget amount greater than $10,000.
8. Order Total Calculation
Scenario: Calculate the total amount of an order based on line items.
csharpCopy codepublic class OrderTotalCalculationPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity order = (Entity)context.InputParameters["Target"];
if (order.LogicalName == "salesorder")
{
QueryExpression query = new QueryExpression("salesorderdetail")
{
ColumnSet = new ColumnSet("extendedamount")
};
query.Criteria.AddCondition("salesorderid", ConditionOperator.Equal, order.Id);
EntityCollection orderDetails = service.RetrieveMultiple(query);
decimal totalAmount = 0;
foreach (Entity detail in orderDetails.Entities)
{
if (detail.Attributes.Contains("extendedamount"))
{
totalAmount += ((Money)detail["extendedamount"]).Value;
}
}
order["totalamount"] = new Money(totalAmount);
}
}
}
}
Explanation: This plugin calculates the total amount of an order by summing up the extended amounts of all related order details.
9. Task Creation on Case Creation
Scenario: Automatically create a follow-up task when a case is created.
csharpCopy codepublic class CreateTaskOnCaseCreation : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity caseEntity = (Entity)context.InputParameters["Target"];
if (caseEntity.LogicalName == "incident")
{
Entity task = new Entity("task");
task["subject"] = "Follow up on case: " + caseEntity["title"];
task["scheduledstart"] = DateTime.Now.AddDays(1);
task["scheduledend"] = DateTime.Now.AddDays(1);
task["regardingobjectid"] = new EntityReference("incident", caseEntity.Id);
service.Create(task);
}
}
}
}
Explanation: This plugin creates a follow-up task with a due date one day after the creation of a new case.
10. Contact Birthday Reminder
Scenario: Send a reminder email when a contact’s birthday is approaching.
csharpCopy codepublic class BirthdayReminderPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity contact = (Entity)context.InputParameters["Target"];
if (contact.LogicalName == "contact" && contact.Attributes.Contains("birthdate"))
{
DateTime birthDate = (DateTime)contact["birthdate"];
if (birthDate.Month == DateTime.Now.Month && birthDate.Day == DateTime.Now.Day + 7)
{
Entity email = new Entity("email");
email["subject"] = "Upcoming Birthday Reminder";
email["description"] = "Reminder: " + contact["fullname"] + "'s birthday is coming up in a week.";
email["to"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("contact", contact.Id) } });
email["from"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("systemuser", context.UserId) } });
service.Create(email);
}
}
}
}
}
Explanation: This plugin sends an email reminder one week before a contact’s birthday.
11. Lead Scoring
Scenario: Automatically score leads based on attributes.
public class LeadScoringPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity lead = (Entity)context.InputParameters["Target"];
if (lead.LogicalName == "lead")
{
int score = 0;
if (lead.Attributes.Contains("budgetamount") && ((Money)lead["budgetamount"]).Value > 5000)
{
score += 10;
}
if (lead.Attributes.Contains("industrycode") && (OptionSetValue)lead["industrycode"] == new OptionSetValue(1))
{
score += 20;
}
lead["leadscore"] = score;
}
}
}
}
Explanation: This plugin assigns a score to a lead based on their budget amount and industry code.
12. Update Parent Account Address
Scenario: Update parent account address when a child account’s address changes.
public class UpdateParentAccountAddress : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity childAccount = (Entity)context.InputParameters["Target"];
if (childAccount.LogicalName == "account" && childAccount.Attributes.Contains("parentaccountid"))
{
Guid parentId = ((EntityReference)childAccount["parentaccountid"]).Id;
Entity parentAccount = new Entity("account", parentId);
if (childAccount.Attributes.Contains("address1_city"))
{
parentAccount["address1_city"] = childAccount["address1_city"];
}
if (childAccount.Attributes.Contains("address1_stateorprovince"))
{
parentAccount["address1_stateorprovince"] = childAccount["address1_stateorprovince"];
}
service.Update(parentAccount);
}
}
}
}
Explanation: This plugin updates the parent account’s address fields when the child account’s address changes.
13. Appointment Reminder
Scenario: Send an email reminder for upcoming appointments. Explanation: This plugin sends a reminder email for appointments scheduled for the next day.
public class AppointmentReminderPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity appointment = (Entity)context.InputParameters["Target"];
if (appointment.LogicalName == "appointment" && appointment.Attributes.Contains("scheduledstart"))
{
DateTime startTime = (DateTime)appointment["scheduledstart"];
if (startTime.Date == DateTime.Today.AddDays(1))
{
Entity email = new Entity("email");
email["subject"] = "Appointment Reminder";
email["description"] = "Reminder: You have an appointment scheduled for " + startTime.ToString("f") + ".";
email["to"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("contact", appointment.Id) } });
email["from"] = new EntityCollection(new List<Entity> { new Entity("activityparty") { ["partyid"] = new EntityReference("systemuser", context.UserId) } });
service.Create(email);
}
}
}
}
}
14. Case Resolution
Scenario: Automatically close related tasks when a case is resolved. Explanation: This plugin closes all related tasks when a case is resolved.
public class CaseResolutionPlugin : IPlugin
{
public void Execute(IServiceProvider serviceProvider)
{
IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext));
IOrganizationServiceFactory serviceFactory = (IPluginExecutionContext)serviceProvider.GetService(typeof(IOrganizationServiceFactory));
IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId);
if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity)
{
Entity caseEntity = (Entity)context.InputParameters["Target"];
if (caseEntity.LogicalName == "incident" && caseEntity.Attributes.Contains("statecode") && ((OptionSetValue)caseEntity["statecode"]).Value == 1)
{
QueryExpression query = new QueryExpression("task")
{
ColumnSet = new ColumnSet("activityid")
};
query.Criteria.AddCondition("regardingobjectid", ConditionOperator.Equal, caseEntity.Id);
EntityCollection tasks = service.RetrieveMultiple(query);
foreach (Entity task in tasks.Entities)
{
task["statecode"] = new OptionSetValue(1); // Completed
task["statuscode"] = new OptionSetValue(5); // Example status for completed
service.Update(task);
}
}
}
}
}