RetrieveMultiple Plugin Code to Change View using normal view and Advance Find Views in Dynamics 365 power apps
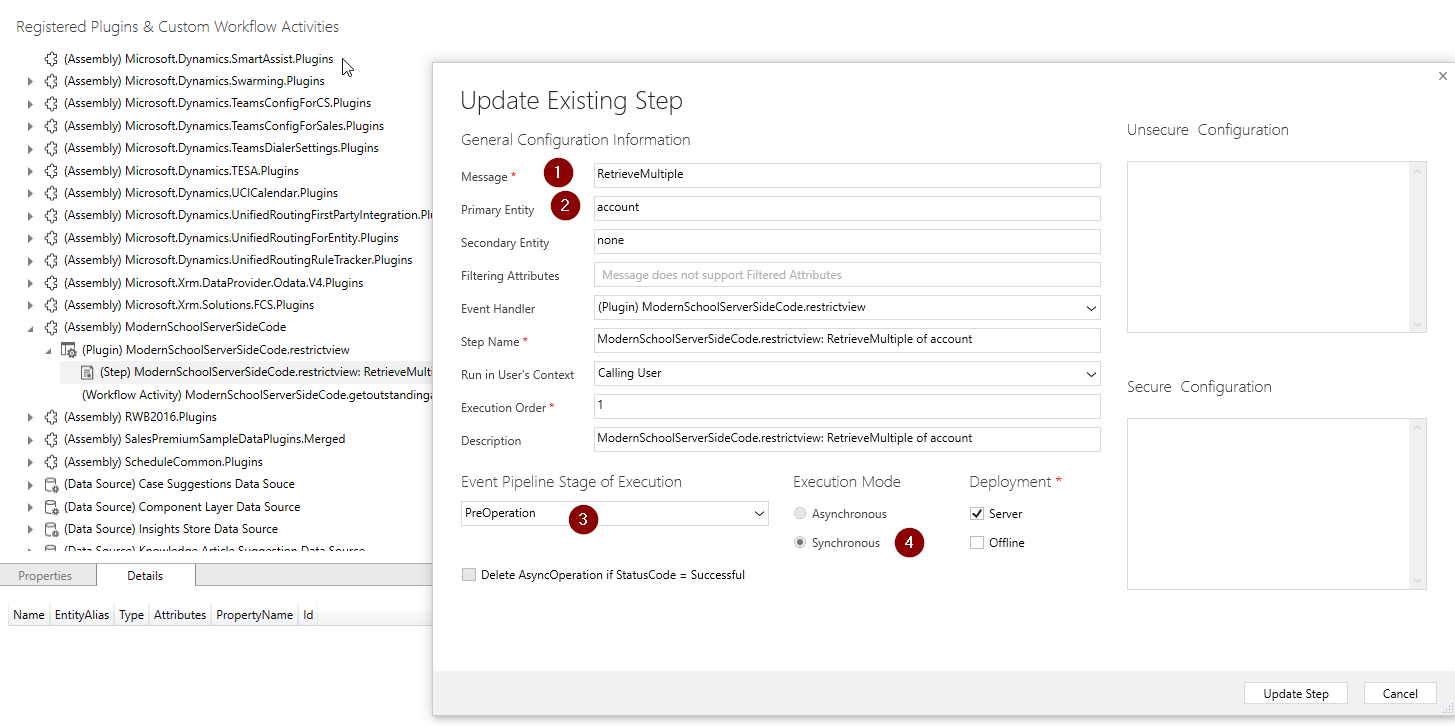
You can use retrievemultiple message if you want to manipulate the records displayed in a view in power apps views or advance find views.
The below plugin code will only display competitor relationship type accounts records if the current user is a IT User else it will display all other account records without relationship type competitor.
using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Xml.Linq; namespace ModernSchoolServerSideCode { public class restrictview : IPlugin { public void Execute(IServiceProvider serviceProvider) { // Obtain the execution context from the service provider. IPluginExecutionContext context = (IPluginExecutionContext)serviceProvider.GetService(typeof(IPluginExecutionContext)); // Get a reference to the Organization service. IOrganizationServiceFactory factory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = factory.CreateOrganizationService(context.UserId); // Get a reference to the tracing service. ITracingService tracingService = (ITracingService)serviceProvider.GetService(typeof(ITracingService)); Guid userID = context.InitiatingUserId; if (context.Mode == 0 && context.Stage == 20 && context.MessageName.Equals("RetrieveMultiple")) { // The InputParameters collection contains all the data passed in the message request. if (context.InputParameters.Contains("Query")) { if (context.InputParameters["Query"] is FetchExpression) // Normal Viw { // Get the QueryExpression from the property bag FetchExpression objFetchExpression = (FetchExpression)context.InputParameters["Query"]; XDocument fetchXmlDoc = XDocument.Parse(objFetchExpression.Query); //The required entity element var entityElement = fetchXmlDoc.Descendants("entity").FirstOrDefault(); var entityName = entityElement.Attributes("name").FirstOrDefault().Value; if (entityName == "account") { //Get all filter elements var filterElements = entityElement.Descendants("filter"); //Find any existing relationshiptype conditions var relationshiptypeConditions = from c in filterElements.Descendants("condition") where c.Attribute("attribute").Value.Equals("customertypecode") select c; if (relationshiptypeConditions.Count() > 0) { tracingService.Trace("Removing existing statecode filter conditions."); //Remove relationshiptype conditions relationshiptypeConditions.ToList().ForEach(x => x.Remove()); } if (UserHasRole(userID, "IT User", service) == true) { //Add the condition you want in a new filter entityElement.Add( new XElement("filter", new XElement("condition", new XAttribute("attribute", "customertypecode"), new XAttribute("operator", "eq"), //equal new XAttribute("value", "1") //comp ) ) ); objFetchExpression.Query = fetchXmlDoc.ToString(); } else { //Add the condition you want in a new filter entityElement.Add( new XElement("filter", new XElement("condition", new XAttribute("attribute", "customertypecode"), new XAttribute("operator", "ne"), //equal new XAttribute("value", "1") //comp ) ) ); objFetchExpression.Query = fetchXmlDoc.ToString(); } } } else if (context.InputParameters["Query"] is QueryExpression) // Advance Find { QueryExpression objQueryExpression = (QueryExpression)context.InputParameters["Query"]; if (objQueryExpression.EntityName.Equals("account")) { tracingService.Trace("Query on Account confirmed"); //Recursively remove any conditions referring to the relationship type column foreach (FilterExpression fe in objQueryExpression.Criteria.Filters) { //Remove any existing criteria based on relationship column RemoveAttributeConditions(fe, "customertypecode", tracingService); } //Define the filter var relationshipCodeFilter = new FilterExpression(); if (UserHasRole(userID, "IT User", service) == true) { relationshipCodeFilter.AddCondition("customertypecode", ConditionOperator.Equal, 1); //Add it to the Criteria objQueryExpression.Criteria.AddFilter(relationshipCodeFilter); } else { relationshipCodeFilter.AddCondition("customertypecode", ConditionOperator.NotEqual, 1); //Add it to the Criteria objQueryExpression.Criteria.AddFilter(relationshipCodeFilter); } } } } } } /// <summary> /// Removes any conditions using a specific named column /// </summary> /// <param name="filter">The filter that may have a condition using the column</param> /// <param name="attributeName">The name of the column that should not be used in a condition</param> /// <param name="tracingService">The tracing service to use</param> private void RemoveAttributeConditions(FilterExpression filter, string attributeName, ITracingService tracingService) { List<ConditionExpression> conditionsToRemove = new List<ConditionExpression>(); foreach (ConditionExpression ce in filter.Conditions) { if (ce.AttributeName.Equals(attributeName)) { conditionsToRemove.Add(ce); } } conditionsToRemove.ForEach(x => { filter.Conditions.Remove(x); tracingService.Trace("Removed existing relationshipetype filter conditions."); }); foreach (FilterExpression fe in filter.Filters) { RemoveAttributeConditions(fe, attributeName, tracingService); } } /// <summary> /// Validate the current user is having a specific role /// </summary> /// <param name="userID"></param> /// <param name="RoleName"></param> /// <param name="service"></param> /// <returns></returns> private static bool UserHasRole(Guid userID, string RoleName, IOrganizationService service) { bool hasRole = false; QueryExpression qe = new QueryExpression("systemuserroles"); qe.Criteria.AddCondition("systemuserid", ConditionOperator.Equal, userID); LinkEntity link = qe.AddLink("role", "roleid", "roleid", JoinOperator.Inner); link.LinkCriteria.AddCondition("name", ConditionOperator.Equal, RoleName); EntityCollection results = service.RetrieveMultiple(qe); hasRole = results.Entities.Count > 0; return hasRole; } } }
This plugin must be registered in RetriveMultiple message and account entity with Pre-Operation stage. Also synchronous type.
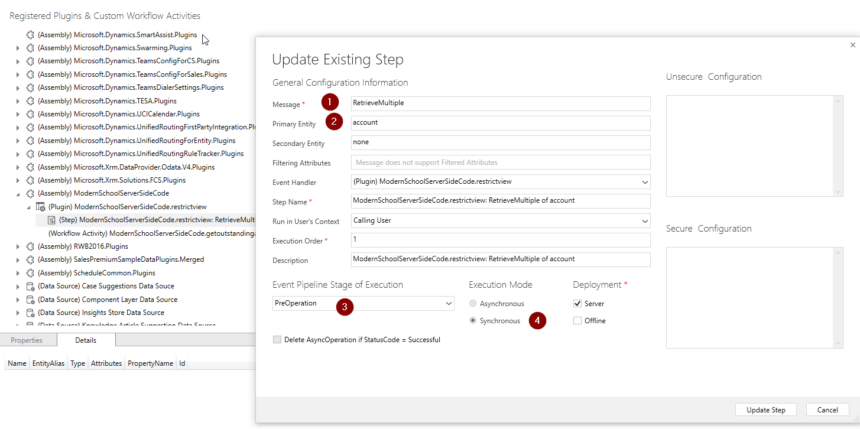
Hope this helps.
Don’t miss the chance to participate in the upcoming Internship Program which will be done using Microsoft Dot Net Web Development Full Stack Technology. The new batch will be starting from May 20, 2024. We will have most experienced trainers for you to successfully complete the internship with live project experience.
Why to choose Our Internship Program?
Industry-Relevant Projects
Tailored Assignments: We offer projects that align with your academic background and career aspirations.
Real-World Challenges: Tackle industry-specific problems and contribute to meaningful projects that make a difference.
Professional Mentorship
Guidance from Experts: Benefit from one-on-one mentorship from seasoned professionals in your field.
Career Development Workshops: Participate in workshops that focus on resume building, interview skills, and career planning.
Networking Opportunities
Connect with Industry Leaders: Build relationships with professionals and expand your professional network.
Peer Interaction: Collaborate with fellow interns and exchange ideas, fostering a supportive and collaborative environment.
Skill Enhancement
Hands-On Experience: Gain practical skills and learn new technologies through project-based learning.
Soft Skills Development: Enhance communication, teamwork, and problem-solving skills essential for career success.
Free Demo Class Available