Sample code for Plugin using Plugin Images in Dynamics 365 or Power Apps Dataverse
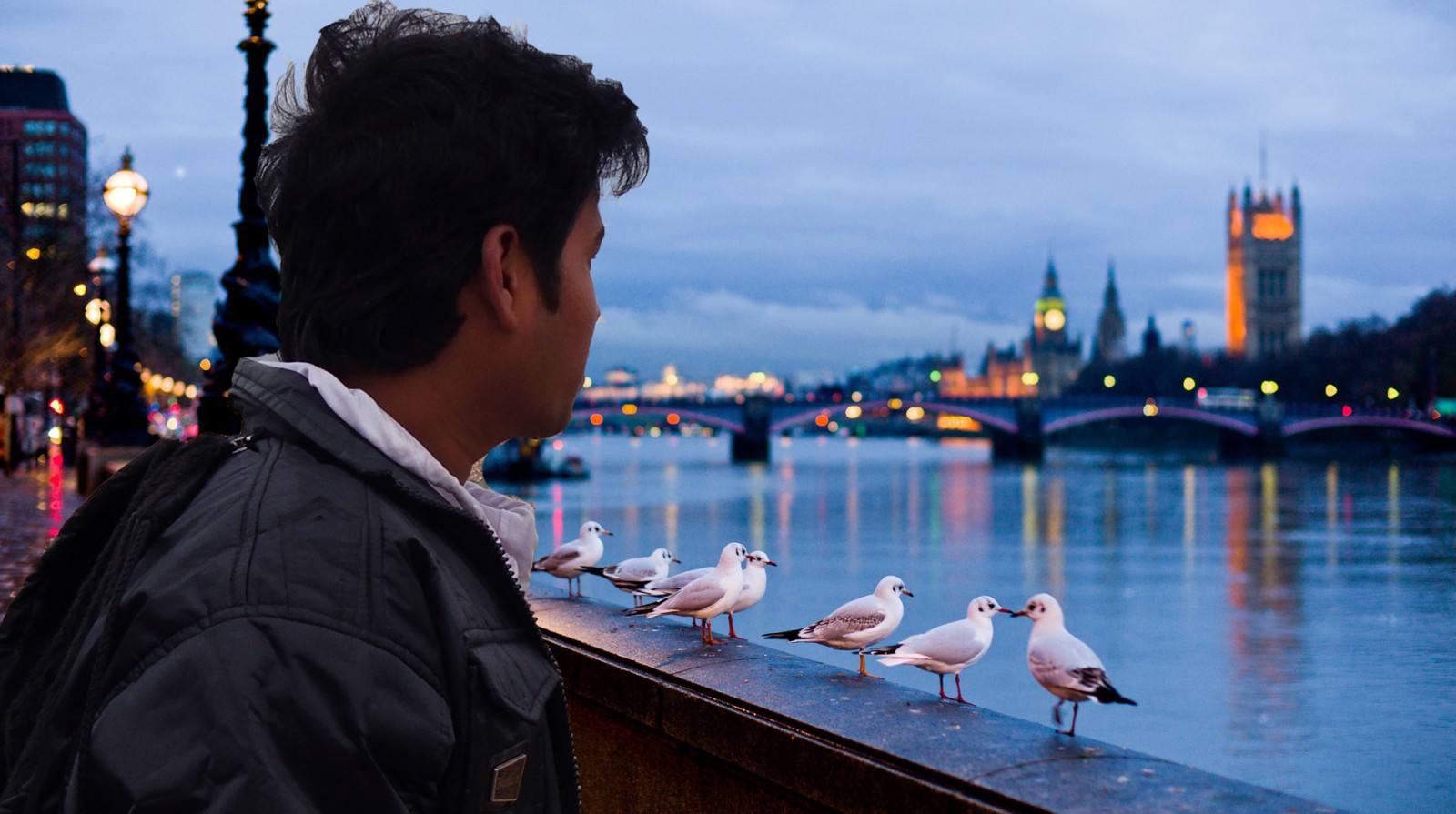
Here sample code given for Plugin using Plugin Images.
using Microsoft.Xrm.Sdk; using Microsoft.Xrm.Sdk.Query; using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace MetroBankProject { public class restrictlessterm : IPlugin { public void Execute(IServiceProvider serviceProvider) { // Obtain the execution context from the service provider. IPluginExecutionContext context = (IPluginExecutionContext) serviceProvider.GetService(typeof(IPluginExecutionContext)); // Obtain the organization service reference which you will need for // web service calls. IOrganizationServiceFactory serviceFactory = (IOrganizationServiceFactory)serviceProvider.GetService(typeof(IOrganizationServiceFactory)); IOrganizationService service = serviceFactory.CreateOrganizationService(context.UserId); // The InputParameters collection contains all the data passed in the message request. if (context.InputParameters.Contains("Target") && context.InputParameters["Target"] is Entity) { Entity entity = (Entity)context.InputParameters["Target"]; if (entity.LogicalName.ToLower() == "hdfc_investment") { //core logic Entity preentityImage = (Entity)context.PreEntityImages["myPreImage"]; var preTerm = preentityImage.Attributes["hdfc_totalterminmonths"]; var postTerm = entity.Attributes["hdfc_totalterminmonths"]; if (Convert.ToInt32(postTerm) > Convert.ToInt32(preTerm)) { throw new InvalidPluginExecutionException("You cannot change term from lower to higher."); } else { var fetchXML = @"<fetch version='1.0' output-format='xml-platform' mapping='logical' distinct='false'> <entity name='hdfc_emiinfo'> <attribute name='hdfc_emiinfoid' /> <attribute name='hdfc_name' /> <attribute name='createdon' /> <order attribute='hdfc_name' descending='false' /> <filter type='and'> <condition attribute='hdfc_parentinvestment' operator='eq' uitype='hdfc_investment' value='{0}' /> </filter> </entity> </fetch>"; fetchXML = string.Format(fetchXML, entity.Id); EntityCollection ec = service.RetrieveMultiple(new FetchExpression(fetchXML)); for (int i = 0; i < ec.Entities.Count; i++) { service.Delete("hdfc_emiinfo", ec.Entities[i].Id); } var amountofinvestment = ((Money)preentityImage.Attributes["hdfc_amount"]).Value; //recreate new EMIs for (int k = 0; k < Convert.ToInt32(postTerm); k++) { // create EMI Info Entity entEMI = new Entity(); entEMI.LogicalName = "hdfc_emiinfo"; entEMI["hdfc_name"] = "Installmnt-" + (k + 1); entEMI["hdfc_parentinvestment"] = new EntityReference(entity.LogicalName, entity.Id); entEMI["hdfc_emiamount"] = new Money(amountofinvestment / Convert.ToInt32(postTerm)); entEMI["hdfc_emiduedate"] = DateTime.Now.AddMonths(k); service.Create(entEMI); } } } } } } }
Hope this helps.