Get and Set Owner Field Using Xrm.WebApi (Applicable to any Lookup Fields)
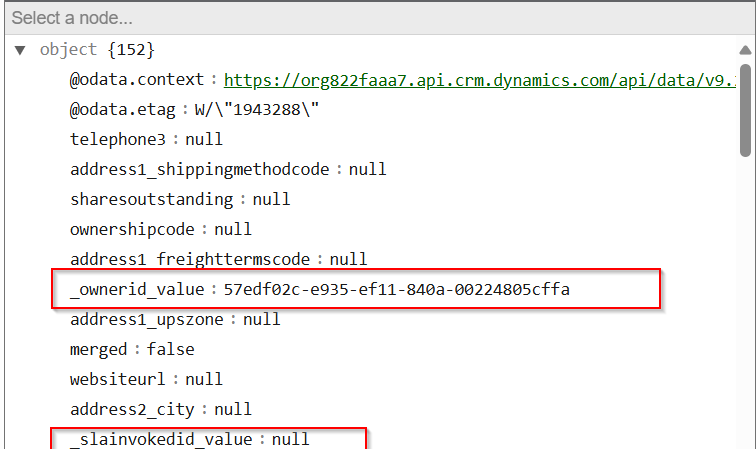
In your Project requirement, you may need to fetch Owner lookup field or any custom or out-of-the-box lookup fields using JavaScript XRM WEB API in Dataverse. Its not straight forward to retrieve lookup fields so you need to follow some steps.
dont use directly the logical name rather use : _<lookupfieldlogicalname>_value
For Example if your lookup field logical name is ownerid then in Xrm.WebApi retrieve call pass as _ownerid_value
If logical name of the lookup field is future_reason then pass _future_reason_value
Here is given a full code how you can call Xrm WebApi for lookup field Owner.
Xrm.WebApi.retrieveRecord("account", "GUID_OF_RECORD", "?$select=_ownerid_value")
.then(function (result) {
console.log("Owner ID: ", result._ownerid_value);
console.log("Owner Name: ", result["_ownerid_value@OData.Community.Display.V1.FormattedValue"]);
console.log("Owner Type: ", result["_ownerid_value@Microsoft.Dynamics.CRM.lookuplogicalname"]);
})
.catch(function (error) {
console.log("Error retrieving owner: ", error.message);
});
Explanation:
result._ownerid_value
→ Retrieves the GUID of the Owner.result["_ownerid_value@OData.Community.Display.V1.FormattedValue"]
→ Retrieves the Name of the Owner.result["_ownerid_value@Microsoft.Dynamics.CRM.lookuplogicalname"]
→ Retrieves the Entity Type (e.g.,"systemuser"
or"team"
).
Clue:
If you want to check what name you should pass for a lookup field, first call Xrm WebAPI without any column selection like below. then on response object you can see all fields returned and you an verify the lookup fields in response.
Xrm.WebApi.retrieveRecord("account", "GUID_OF_RECORD")
.then(function (result) {
console.log("Owner ID: ", result._ownerid_value);
console.log("Owner Name: ", result["_ownerid_value@OData.Community.Display.V1.FormattedValue"]);
console.log("Owner Type: ", result["_ownerid_value@Microsoft.Dynamics.CRM.lookuplogicalname"]);
})
.catch(function (error) {
console.log("Error retrieving owner: ", error.message);
});
You will see this kind of response and once you understand what name it returns, you can specify the columns in the Xrm WebApi query.
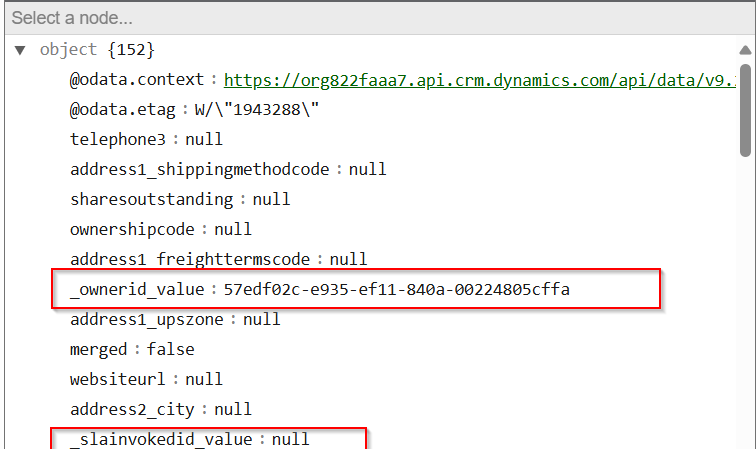
If you want to get its name then you have to Formatted values.
This is sample code.
for (var i = 0; i < result.entities.length; i++) {
var p = result.entities[0];
if (p != null) {
var lookup = new Array();
lookup[0] = new Object;
lookup[0].id = p["_ownerid_value"];
lookup[0].name = p["_ownerid_value@OData.Community.Display.V1.FormattedValue"];
lookup[0].entityType = p["_ownerid_value@Microsoft.Dynamics.CRM.lookuplogicalname"];
Xrm.Page.getAttribute("ownerid").setValue(lookup);
}
Iwe can set it on our field on the form like below:
Xrm.WebApi.retrieveMultipleRecords("systemuser", "?$select=fullname,ownerid&$filter=fullname eq 'sanjay'").then(
function success(result) {
for (var i = 0; i < result.entities.length; i++) {
var p = result.entities[0];
if (p != null) {
var lookup = new Array();
lookup[0] = new Object;
lookup[0].id = p["_ownerid_value"];
lookup[0].name = p["fullname"];
lookup[0].entityType = p["_ownerid_value@Microsoft.Dynamics.CRM.lookuplogicalname"];
Xrm.Page.getAttribute("ownerid").setValue(lookup);
}
break; // We will grab the first one
}
},
function(error) {
alert("Error: " + error.message);
}
);
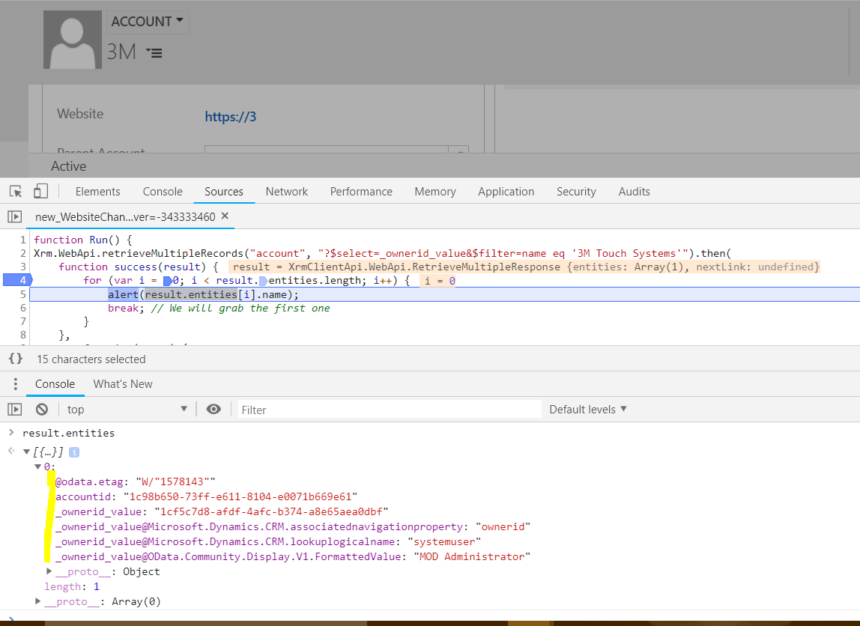
Hope It helps.